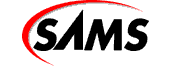
Teach Yourself Borland Delphi 4 in 21 Days
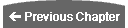
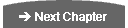
- A -
Answers to the Quiz Questions
This appendix provides the answers to the quiz sections at the end of each chapter.
Day 1
- 1. What is the filename extension of a Pascal unit?
- .pas
- 2. What is the name of the keyword that marks the section in which variables
are declared?
- var
- 3. What does the IntToStr function do?
- The IntToStr function converts an integer value into a Pascal string.
- 4. What is the purpose of the uses list in a Pascal unit?
- The uses list in a Pascal unit lists the units that this unit is dependent on.
The compiler needs to be able to see those units in order to compile the current
unit.
- 5. Are the following two declarations different? Why or why not?
var
top : Integer;
Top : Integer;
- The declarations are identical because the Pascal language is not case sensitive.
- 6. How do you concatenate Pascal strings?
- By using the + operator. (You can also use the StrCat function for null-terminated
strings.)
- 7. How can you embed a control character in a string?
- By using the # symbol followed by the ASCII value of the character you want to
embed.
- 8. What is the maximum length of a short string?
- 255 characters.
- 9. Look at this line of code:
MyArray : array [0..10] of Byte;
- How many bytes can this array hold?
- 11 bytes (0 through 10).
- 10. What is the index number of the first element of an array, 0 or 1?
- That depends on how the array is declared. This array has a base index of 0:
Array1 : array [0..9] of Integer;
- This array, however, has a base index of 1:
Array2 : array [1..10] of Integer;
Day 2
- 1. What statements are executed in the event an if expression evaluates
to True?
- The statement immediately following the if statement. If a code block follows
an if statement, the entire code block will be executed.
- 2. How many return values can a function return?
- One. On the other hand, if you implement variable parameters, a function can
effectively return more than one value.
- 3. Besides syntax, what is the difference between a while loop and a repeat
loop?
- A while loop checks the conditional expression at the beginning of the loop.
A repeat loop checks the conditional expression at the end of the loop.
- 4. What do the Break and Continue procedures do?
- The Break procedure is used to break out of a loop. The statement following the
body of the loop will be executed after a call to Break. The Continue procedure forces
program execution back to the top of the loop.
- 5. What is a global variable?
- A variable that is in scope anywhere in the program. It can be accessed by any
function in the program.
- 6. Can a record contain a mixture of data types (Char, Integer, Word,
and so on)?
- Yes, a structure can contain any number and type of data members.
- 7. How do you access the members of a record?
- With the dot operator (.). Here's an example:
record.LastName = "Noble";
- 8. How many functions or procedures can a program have?
- There is no practical limit to the number of functions or procedures a program
can have.
- 9. Can a function call another function or procedure?
- Yes, functions and procedures can, and often do, call other functions or procedures.
- 10. Is it legal to have arrays of records?
- Yes, you can have arrays of records just as easily as you can have arrays of
integers, bytes, or strings.
Day 3
- 1. How do you clear a set of all values?
- By assigning an empty set constructor to the set--like this:
Font.Style := [];
- 2. What is the purpose of having private fields and methods?
- Private data members protect data from being modified directly by users of the
class. Private data members can be modified through public member functions or properties,
but not directly.
- 3. How can you keep fields private and yet enable users to read and set
their values?
- By using methods or properties.
- 4. When is a class's destructor called?
- The destructor is called when the object is destroyed.
- 5. What does it mean to override a method of the base class?
- To override a method means to replace a method in the base class with a method
in your derived class. The new method must have the exact same name, parameters,
and return type to override the base class method.
- 6. How can you override a base class method and still get the benefit
of the operation the base class method performs?
- Call the base class function from within the overridden function:
procedure MyClass.DoIt;
begin
Inherited DoIt;
{ do some other stuff }
end;
- 7. What operator is used to dereference a pointer?
- The pointer operator (^).
- 8. Can a class contain other class instances as fields?
- Yes. It's very common.
- 9. What keyword is used to specify a pointer that has no value?
- The nil keyword.
- 10. What is the as keyword used for?
- To cast a pointer from a derived type to an ancestor type, or vice versa.
Day 4
- 1. How do you invoke the main window's Customize dialog box?
- Right-click any toolbar and choose Customize from the context menu.
- 2. When you have the Customize dialog box open, how do you add buttons
to a toolbar?
- Simply drag an item from the Commands page to a toolbar and drop it where you
want the item to appear on the toolbar.
- 3. How do you remove buttons from a toolbar?
- Drag unwanted buttons off the bottom of the toolbar and drop them.
- 4. What's the easiest way to place multiple components of the same type
on a form?
- Hold the Shift key when you click the component in the Component palette. Each
time you click on the form, a new component will be placed.
- 5. What's the easiest way to place a component in the center of the form?
- Double-click the component's button in the Component palette.
- 6. List the file types needed to build an application in Delphi.
- The .dpr, .pas, and .dfm files.
- 7. What VCL method do you use to display a form modelessly?
- The Show method.
- 8. What VCL method do you use to display a form modally?
- The ShowModal method.
- 9. How can you attach an event to an event handler that has been previously
defined?
- In the Object Inspector, switch to the Events page. In the value column next
to the event, click the drop-down arrow button. A list of compatible event handlers
is displayed. Choose one.
- 10. When using the Object Inspector, how can you enumerate the choices
for a particular property?
- Double-click the value column next to the property name in the Object Inspector.
Each time you double-click, the value changes to the next item in the list.
Day 5
- 1. Are all components visible at design time?
- No. Only visual components can be seen at design time.
- 2. Is the OpenDialog component a visual component or a nonvisual component?
- It's a nonvisual component. Although it is displayed at runtime, it is considered
nonvisual because it is not visible at design time.
- 3. What is the name of the VCL class that represents a Delphi form?
- TForm.
- 4. Do all versions of Delphi ship with the same set of components?
- No. The Professional version comes with more components than the Standard version.
Likewise, the Client/Server version comes with more components than does the Professional
version.
- 5. Are all VCL classes ultimately derived from TObject?
- Yes.
- 6. Name one nonvisual VCL component.
- TOpenDialog, TSaveDialog, TRegistry, TColorDialog, TTimer, TImageList, TFontDialog,
and many more, are all nonvisual VCL components.
- 7. Do all components share certain common properties?
- Yes. All components are ultimately derived from TComponent, so they all have
the properties found in TComponent, such as Name and Owner.
- 8. Name two common properties that all visual components share.
- Common properties that all visual components share include Top, Left, Owner,
Parent, Width, Height, and so on.
- 9. Can two or more components share the same event handler?
- Yes.
- 10. What is the VCL terminology for a Windows device context? What is
the name of the VCL class that encapsulates device contexts?
- A canvas is a Windows device context. VCL encapsulates device contexts through
the TCanvas class.
Day 6
- 1. When do you use Ctrl+drag in selecting components?
- When selecting components that are children of another component (components
on a panel, for example).
- 2. What significance does the first component selected have when aligning
a group of components?
- It is the anchor component. It retains its position, and all other components
are aligned to it.
- 3. What is the quickest method to select a group of components?
- Drag a bounding rectangle around (or just touching) them.
- 4. How can you make a group of components all have the width of the group's
widest component?
- Select all the components you want to modify. Then choose Edit | Size from the
main menu and choose the Grow to Largest radio button.
- 5. What happens when you double-click a component on a form?
- The default event handler for that component is displayed in the Code Editor.
In the case of many components, the OnClick event handler will be displayed. In some
special cases (such as the Image component), a dialog box is displayed.
- 6. What does the Align property's alClient option do?
- It forces the component to fill the entire client area of its parent, regardless
of how the parent (usually a form) is sized.
- 7. What does the ellipsis following a menu item mean?
- Traditionally, it means that choosing that menu item will result in a dialog
being displayed.
- 8. What two ways can you move a menu item?
- In the Menu Designer, you can drag the menu to a new location or you can use
cut and paste.
- 9. How do you add menu accelerators to menu items?
- When typing the caption for the menu item, add the ampersand (&) before the
shortcut key you choose as the shortcut for that menu item. For example, the Caption
for the File | Exit menu item would read E&xit.
- 10. How do you initially disable a menu item?
- Set its Enabled property to False.
Day 7
- 1. Can you change the Name property of a component at runtime?
- Yes, but it's a very bad idea.
- 2. What property is used to enable and disable controls?
- The Enabled property.
- 3. How can you tell at runtime that a button is disabled?
- Its text is grayed out.
- 4. What is the difference between the long hint and the short hint?
- The long hint is used for the status bar text, and the short hint is used for
the tooltip text.
- 5. Name three of the four methods that can be used to tell a control to
repaint itself.
- Invalidate, Repaint, Refresh, and Update.
- 6. How many types of combo boxes are there?
- Three: simple, drop-down, and drop-down list.
- 7. How is the ModalResult property used for button components?
- When a button with a ModalResult property set to a whole number is clicked, the
form will close. The value of the ModalResult property for the button clicked will
be the return value from the ShowModal method.
- 8. What component is often used as a container for other components?
- The Panel component. Several others qualify, too.
- 9. What is the return value from the Execute method for an OpenDialog
component if the user clicks OK to close the dialog box?
- true.
- 10. How do you make the SaveDialog component into a Save As dialog box?
- Just change its Title property to Save As.
Day 8
- 1. When do you use the Inherit option when selecting an object in the
Object Repository?
- Use the Inherit option when you want all the features of the base object and
you want the inherited object to change if the base object changes.
- 2. What is the procedure for saving a project to the Object Repository?
- To save a project to the Object Repository, choose Project|Add to Repository
from the main menu.
- 3. What happens to inherited forms when you change the base form?
- When you change the base form, all the inherited forms change to reflect the
change made to the base form.
- 4. Where in the form's class declaration do you place user method declarations?
- You place user method declarations in the private or public sections of the class
declaration. Never place user declarations in the Delphi-managed section of the class
declaration (unless you know what you are doing).
- 5. Where do you place the method definition (the method itself) when you
add your own methods to Delphi code?
- In the implementation section of the unit.
- 6. How can you determine who wrote a particular object in the Object Repository?
- You can tell who wrote an object in the Object Repository by switching to the
Details view. The object's author is listed there.
- 7. Where do you add and delete pages in the Object Repository?
- You add or delete pages in the Object Repository from the Object Repository configuration
dialog box (which you get by selecting Tools | Repository from the main menu).
- 8. Is it easier to create a basic application from scratch or by using
the Application Wizard?
- It is easier to create a new application by using the Application Wizard in almost
all cases.
- 9. Which is better for small applications: static linking or dynamic linking
using packages?
- For small applications, static linking is usually better than dynamic linking
(no runtime packages).
- 10. Can you create a resource script file containing a string table with
a text editor?
- Yes, you can easily create a string table with a text editor. You only need to
understand the basic layout of a string table.
Day 9
- 1. How can you quickly switch between a unit's form and source code when
working with Delphi?
- Use the F12 key to quickly switch between the Form Designer and the Code Editor.
- 2. If you remove a file from your project via the Project Manager, is
the file removed from your hard drive?
- No, it is only removed from the project.
- 3. How do you set the main form for an application?
- Go to the Forms page of the Project Options dialog box and select the form you
want to be the main form in the Main form combo box.
- 4. What does it mean if you do not have Delphi Auto-create forms?
- You will have to take the responsibility of creating the forms before using them.
- 5. How do you add new items to your unit using the Code Explorer?
- Right-click and choose New from the Code Explorer context menu. Type in the declaration
for the new item and hit Enter.
- 6. What is the significance of generating debug information for your application?
- When debug information is generated, you will be able to step through your code
during debugging sessions.
- 7. What is the Find in Files option used for?
- Find in Files is used to find text in files.
- 8. What is the keyboard shortcut for saving a file in the Code Editor?
- Ctrl+S (assuming that you're using the default keymapping).
- 9. How do you set a bookmark in an editor window? How many bookmarks are
available?
- Set a bookmark with Ctrl+K+0 through Ctrl+K+9. There are 10 bookmarks available.
- 10. How do you set a file to read-only in the Code Editor?
- Choose Read Only from the Code Editor context menu.
Day 10
- 1. How do you set a breakpoint on a particular code line?
- Click in the gutter (the left margin) on that code line. You can also press F5
or choose Toggle Breakpoint from the Code Editor context menu.
- 2. What is an invalid breakpoint?
- A breakpoint that is inadvertently set on a source code line that generates no
compiled code.
- 3. How do you set a conditional breakpoint?
- Set the breakpoint, choose View|Debug Windows|Breakpoints from the main menu,
click the breakpoint in the Breakpoint List window, and then choose Properties from
the Breakpoint List context menu. Set the condition in the Condition field of the
Edit Breakpoint dialog box.
- 4. How can you change the properties of an item in the Watch List?
- Double-click the item in the Watch List window. The Watch Properties dialog box
is displayed. Modify the properties as needed.
- 5. What's the quickest way to add a variable to the Watch List?
- Click the variable and press Ctrl+F5 (or choose Add Watch at Cursor from the
Code Editor context menu).
- 6. What tool do you use to view the data fields and methods of a class?
- The Debug Inspector is used to view classes and records.
- 7. How do you trace into a method when stepping with the debugger?
- Use F7 or Run|Trace Into to step into a method.
- 8. How can you change the value of a variable at runtime?
- Click the variable and then choose Evaluate/Modify from the Code Editor context
menu (or choose Run|Evaluate/Modify from the main menu). Change the value in the
Evaluate/Modify dialog box.
- 9. How can you send your own messages to the Event Log?
- You can send your own messages to the Event Log by calling the Windows API function,
OutputDebugString.
- 10. What does the Integrated debugging option on the Debugger Options
dialog box do?
- When this option is on and the program is run from the IDE, the program runs
under control of the debugger. When this option is off, the program runs without
using the debugger.
Day 11
- 1. How is the transparent color used for icons and cursors?
- The background shows through wherever the transparent color is used on the icon
or cursor.
- 2. How do you choose a color in the Image Editor?
- Click a color in the color palette.
- 3. How do you select an area on a bitmap to cut or copy?
- Choose the Marquee tool and then drag a rectangle with the mouse. You could also
use Edit|Select All to select the entire image or the Lasso tool.
- 4. What is the maximum number of colors allowed in an Image Editor bitmap?
- 256.
- 5. What is a cursor's hot spot?
- The exact pixel in the cursor that is used to report the screen coordinates to
Windows when the mouse is clicked.
- 6. Can WinSight spy on hidden windows?
- Yes. WinSight shows hidden windows as well as visible windows.
- 7. What's the fastest way to locate a window in the WinSight Window Tree?
- Choose Window|Follow to Focus from the main menu and then click on the window
you want to spy on.
- 8. How can you have your editor files automatically saved each time your
program runs through the debugger?
- Turn on the Editor files option on the Preferences page (Autosave options section)
of the Environment Options dialog box.
- 9. Where do you go to rearrange the contents of the Component Palette?
- Use the Palette page of the Environment Options dialog box.
Day 12
- 1. What component can you use to draw graphics on a form?
- Although you can draw directly on the form's canvas, the PaintBox component enables
you to draw in a predefined area of a form (the area the PaintBox occupies).
- 2. Which TCanvas property controls the fill color of the canvas?
- The Brush property.
- 3. What does a clipping region do?
- The clipping region defines a canvas area within which drawing can take place,
but outside which no drawing takes place. Any drawing outside the clipping region
won't be displayed.
- 4. What function do you use to draw multiple lines of text on a canvas?
- The DrawText function with the DT_WORDBREAK flag.
- 5. What TCanvas method can be used to draw a bitmap with a transparent
background?
- The BrushCopy method.
- 6. Which TCanvas method do you use to copy an entire bitmap to a canvas?
- You can use several, but the easiest and fastest is the Draw method. Others include
BrushCopy, StretchDraw, and CopyRect.
- 7. How do you save a memory bitmap to a file?
- With the SaveToFile method.
- 8. What component do you use to play a wave file?
- The TMediaPlayer component. To play a wave file using the Windows API, use the
PlaySound function.
- 9. What is the TimeFormat property of TMediaPlayer used for?
- The TimeFormat property is used to set the time format based on the media type
being played. Some media types can use several time formats (CD audio, for example).
- 10. Can you record wave audio with the MediaPlayer component?
- Yes, but you have to jump through some hoops.
Day 13
- 1. How do you attach an event handler to a toolbar button's OnClick event?
- In the Object Inspector, click the Event tab. Click the drop-down arrow next
to the OnClick event. Choose an event handler from the list.
- 2. Can you put controls other than buttons on toolbars?
- Yes. You can put virtually any type of component on a toolbar. Combo boxes are
commonly found on toolbars.
- 3. What is the name of the TActionList event you respond to when doing
command enabling?
- The OnUpdate event.
- 4. What does the SimplePanel property of the StatusBar component do?
- It forces the status bar to have a single panel.
- 5. How do you change the status bar text manually?
- For a simple status bar, use the following:
StatusBar.SimpleText := `Text';
- 6. How do you enable and disable menu items and buttons?
- For individual components, set the component's Enabled property to True to enable
the component or False to disable it. For several components that have a common task,
create an Action for that task and set the Action's Enabled property accordingly.
- 7. How do you access the printer in a Delphi application?
- Through the Printer function.
- 8. What method do you call to begin printing with the TPrinter class?
- The BeginDoc method.
- 9. What method of TPrinter do you call when you want to start a new page
when printing?
- The NewPage method.
- 10. How do you change the cursor for a component at runtime?
- Modify the component's Cursor property.
Day 14
- 1. How do you set the help file that your application will use?
- Use the Project Options dialog (Application page) or set the HelpFile property
of the Application object at runtime.
- 2. How do you implement F1 key support for a particular form or dialog
box?
- Just assign a nonzero value to the HelpContext property. Make sure that there
is a corresponding help context ID in the help file and that the help file has been
set for the application.
- 3. What method do you call to display the index for your help file?
- The HelpCommand method.
- 4. What types of objects can an exception raise?
- Any class derived from Exception.
- 5. Is it legal to have more than one except statement following a try
statement?
- No, there can only be one except statement.
- 6. How do you raise an exception?
- With the raise keyword--for example,
raise EMyException.Create(`An error occurred.');
- 7. What is the default value of the TRegistry class RootKey property?
- \HKEY_CURRENT_USER
- 8. Must you call CloseKey when you are done with a key?
- No. The TRegistry destructor will close the key for you. You shouldn't leave
a key open indefinitely, though.
- 9. What is the difference between SendMessage and PostMessage?
- PostMessage posts the message to the Windows message queue and returns immediately.
SendMessage sends the message and doesn't return until the message has been handled.
- 10. What is the name of the VCL method that is used to send a message
directly to a component?
- The Perform method.
Day 15
- 1. What is the base (or parent) interface for all COM interfaces?
- The base interface from which all other interfaces are derived is IUnknown.
- 2. What is a GUID?
- A GUID is a 128-bit integer that uniquely identifies a COM object (interface,
class, or type library).
- 3. What happens when a COM object's reference count reaches 0?
- When a COM object's reference count reaches 0, the COM object is unloaded from
memory by Windows.
- 4. What is the name of the Delphi utility used when working with type
libraries?
- The utility used to modify type libraries is the Type Library Editor.
- 5. How do you create GUIDs when writing COM objects in Delphi?
- You don't have to specifically create GUIDs when creating COM objects in Delphi.
Delphi creates the GUIDs for you automatically (trick question, I know). If you want
to specifically create a GUID in your code, you can press Ctrl+Shift+G in the Code
Editor. Delphi will generate and insert a GUID in your code.
- 6. What do you choose from the Object Repository when creating an ActiveX
from a VCL component?
- To create an ActiveX control from an existing VCL component, choose the ActiveX
Control item in the Object Repository.
- 7. Can you use Delphi-created ActiveX controls in Visual Basic?
- Yes, you can use Delphi ActiveX controls in VB. You must be sure that the ActiveX
contains version information, but otherwise a Delphi ActiveX should work in VB with
no trouble.
- 8. After your ActiveX control is built and registered, how do you install
it to the Delphi Component palette?
- To install an ActiveX control to the Delphi Component palette, choose Component
| Import ActiveX Control from the Delphi main menu.
- 9. How do you unregister an ActiveX that you have created?
- To unregister an ActiveX control, you can either choose Run | Unregister ActiveX
Server from the main menu or you can run the TREGSVR utility on the ActiveX with
the -u switch. You can also uninstall an ActiveX control from the Import ActiveX
dialog box.
- 10. Can you use the ActiveX controls created in Delphi on a Web page?
- Yes. Delphi ActiveX controls are designed to be deployed on a Web page.
Day 16
- 1. What is a local database?
- A database that resides on the user's machine rather than on a database server.
This term usually refers to Paradox or dBASE tables.
- 2. What is the purpose of the BDE?
- The BDE provides your Delphi application access to databases.
- 3. Are a dataset and a table the same thing? If not, explain the difference.
- No, they are not the same thing. A dataset might include an entire table's contents,
or it might contain only a small subset of the table.
- 4. Name one advantage of cached updates.
- Cached updates reduce network traffic, enable you to modify a read-only dataset,
and enable you to make several changes and then either commit or roll back all changes
at once.
- 5. What is a stored procedure?
- An application that acts on a database and resides on a database server.
- 6. What is the purpose of the SQL property of the TQuery component?
- The SQL property contains the SQL statements to execute when the Open or Execute
methods are called.
- 7. Name one reason you might want to use your own TDatabase object instead
of the default.
- To allow automatic login to a database.
- 8. Why would you want to keep a connection to a remote database open even
when you are not currently using the connection?
- To reduce the time required to log in to the database each time a connection
is requested.
- 9. What does the TBatchMove component do?
- TBatchMove enables you to create or modify one dataset with the contents of another
dataset.
- 10. What is a BDE alias?
- A set of parameters that describes a database connection.
Day 17
- 1. What's the fastest and easiest way to create a database form?
- With the Database Form Wizard.
- 2. How do you control the order and number of columns that appear in a
DBGrid component?
- With the Columns Editor. (Right-click a DBGrid component and choose Columns Editor
from the context menu.)
- 3. What component enables you to display a dataset in table format?
- The DBGrid component.
- 4. How can you add or remove buttons from a DBNavigator?
- By modifying the VisibleButtons property.
- 5. What component do you use to display BLOB image data?
- The DBImage component.
- 6. What property is common to all data-aware components?
- The DataSource property (among others).
- 7. What property is used to select the field that a data component is
linked to?
- The DataField property.
- 8. Can you rearrange the columns in a DBGrid component?
- Yes. Use the Columns Editor at design time or drag and drop them at runtime.
- 9. Which component is used to edit and display text fields in a database?
- The DBEdit component.
- 10. What does BLOB stand for?
- Binary large object.
Day 18
- 1. What method do you call to create a database table at runtime?
- CreateTable.
- 2. What does the Edit method of TTable do?
- The Edit method puts the dataset in edit mode so that records can be modified.
- 3. What method do you call when you want to apply the changes made to
a record?
- The Post method.
- 4. How do you create a new data module?
- Through the Object Repository.
- 5. Is a data module a regular form?
- A data module is very similar to a regular form but not the same.
- 6. What method do you call to print a QuickReport?
- The Print method.
- 7. What type of QuickReport band displays the dataset's data?
- A detail band.
- 8. What component is used to display the page number on a report?
- The QRSysData component can display page numbers, the current date, the current
time, and more.
- 9. How can you preview a report at design time?
- Right-click the report and choose Preview from the context menu.
- 10. What is the QRExpr component used for?
- The QRExpr component is used to display the results of an expression (usually
a calculated result).
Day 19
- 1. How do you load a DLL using static loading?
- In your calling application declare any functions or procedures contained in
the DLL using the extern keyword. The DLL will automatically load when the application
starts.
- 2. How do you load a DLL using dynamic loading?
- Use the Windows API function LoadLibrary.
- 3. How do you call a function or procedure from a DLL that has been loaded
statically?
- Call the function or procedure just as you would call a regular function or procedure.
- 4. What steps do you have to take to ensure that a function or procedure
in your DLL can be called from outside the DLL?
- The function or procedure must be exported from the DLL. Place the function or
procedure name in the exports section of the DLL.
- 5. In the case of a DLL that has been dynamically loaded, can you unload
the DLL at any time or only when the program closes?
- You can unload the DLL any time you want (using the FreeLibrary function).
- 6. What must you do to display a Delphi form contained in a DLL from a
non-Delphi program?
- Create an exported function that the calling application can call. In this function,
create the form and display it. Be sure the function is declared with the stdcall
keyword.
- 7. What is the name of the keyword used to declare functions and procedures
imported from a DLL?
- The external keyword.
- 8. How do you add resources to a DLL?
- Link a compiled resource file (.res or .dcr) to the DLL with the $R compiler
directive--for example,
{$R Resources.res}
- 9. Does a resource DLL need to contain code as well as the resources?
- No. A resource DLL doesn't need any code.
- 10. Can a DLL containing resources be loaded statically (when the program
loads)?
- It's possible, but there's rarely any reason to load a resource DLL statically.
You need the return value from LoadLibrary to access resources in the DLL, so you
might as well use dynamic loading for resource DLLs.
Day 20
- 1. Must a property use a write method? Why or why not?
- No. You can use direct access instead.
- 2. Must a property have an underlying data field? Why or why not?
- No, a property doesn't have to have an underlying data field, but most properties
usually do. A property that doesn't store a value is unusual.
- 3. Can you create a component by extending an existing component?
- Absolutely. That's the easiest way to create a new component.
- 4. What happens if you don't specify a write specifier (either a write
method or direct access) in a property's declaration?
- The property becomes read-only.
- 5. What does direct access mean?
- The underlying data member for the property is modified and/or read directly
(no read method and no write method).
- 6. Must your properties have a default value? Why or why not?
- No, default values for properties are optional. Published properties should have
a default value, though. Properties that are string properties cannot have a default
value (and some other types of properties as well).
- 7. Does the default value of the property set the underlying data field's
value automatically?
- No, the default value only displays a value in the Object Inspector at design
time. You must set the underlying data member to the default value in the component's
constructor.
- 8. How do you install a component on the Component Palette?
- Choose Component | Install from the main menu.
- 9. How do you specify the button bitmap that your component will use on
the Component Palette?
- Create a .dcr file with the same name as the component's source. The .dcr should
contain a 24¥24 bitmap with the same name as the component's classname. Be sure
that the .dcr is in the same directory as the .pas file for the component.
- 10. How do you trigger a user-defined event?
- Just call the event after checking whether an event handler exists for the event:
if Assigned(FOnMyEvent) then
FOnMyEvent(Self);
Day 21
- 1. Do Delphi and C++Builder project files have the same filename extension?
- No, Delphi and C++Builder project files do not have the same filename extension.
Delphi projects have a .dpr extension and C++Builder projects have a .bpr extension.
- 2. Do Delphi and C++Builder form files have the same filename extension?
- Yes. Both Delphi and C++Builder have .dfm filename extensions.
- 3. Can you use packages from third-party component vendors in both Delphi
and C++Builder?
- In most cases, you can use packages from third-party component vendors in both
Delphi and C++Builder. In some cases, the packages will have to be rebuilt with C++Builder.
Ask the component vendor for packages compatible with C++Builder.
- 4. Can you open a Delphi form file in C++Builder?
- Yes. You cannot edit the form (add or remove components), but you can view the
form.
- 5. Can you edit a Delphi form file using the C++Builder Form Designer?
- No, you cannot edit a Delphi form file using the C++Builder Form Designer. However,
you can edit the Delphi form as text by choosing View As Text from the C++Builder
context menu.
- 6. Can you use a C++Builder source unit in Delphi?
- With the current version of Delphi, you cannot use a C++Builder source unit in
Delphi.
- 7. Which is better, Delphi or C++Builder?
- Neither Delphi or C++Builder is better. Both have their strengths. It depends
largely on whether you prefer Pascal or C++.
Bonus Day
- 1. What control do you use to display Web pages?
- The THMTL control.
- 2. What control do you use to connect to newsgroups?
- The TNMNNTP control.
- 3. What is the name of the method used to display an HTML document with
the THTML control?
- RequestDoc.
- 4. What event is generated when an HTML document has completed loading?
- The OnEndRetrieval event.
- 5. What control do you use to send email messages?
- The TNMSMTP control.
- 6. What control do you use to retrieve email messages?
- The TNMPOP3 control.
- 7. What is the name of the method used to send mail with the TNMSMTP control?
- SendMail.
- 8. Can you freely distribute the Internet Explorer ActiveX control?
- No, you must obtain a license from Microsoft to distribute the Internet Explorer
ActiveX control.
- 9. What is the name of the utility used to register ActiveX controls?
- TREGSVR.EXE.
- 10. What company provides the bulk of the Internet controls that come
with Delphi?
- NetMasters.
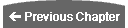
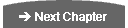
© Copyright, Macmillan Computer Publishing. All
rights reserved.